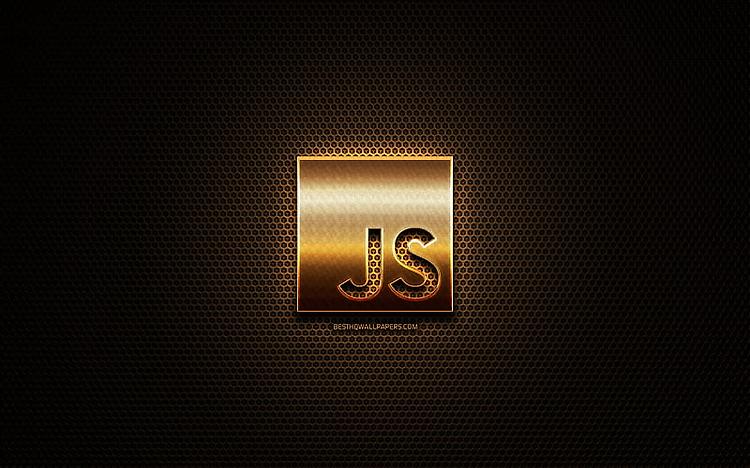
클래스를 이용한 모듈화개발/JavaScript2023. 7. 1. 20:21
Table of Contents
*부족한 내용들은 천천히 보충해나갈것임.
JS에서 Class를 활용해서 모듈화해서
각각의 Class에 해당하는 기능들을 만들고 조합하는 방법에 대해 짧게 기록해보려 한다.
Q. 자바도 아닌데 자바스크립트에서 왜 Class 를 활용하나?
A. Class를 활용하면 객체의 상태와 메서드를 하나의 단위로 묶어서 관리할 수 있고
이를 통해 유지보수성이 좋아지고 중복 코드가 줄어들며 코드의 가독성도 높일 수 있다.
즉, 객체의 청사진을 만들어 속성과 메서드를 정의한 다음 필요할 때 인스턴스화 할 수 있고
이를 통해 코드베이스 전체에서 해당 객체를 쉽게 관리하고 조작할 수 있다.
예를 들어, 아래와 같이 Person 클래스와 Phone 클래스가 있다고 가정해보자
class Person {
constructor(name, age) {
this.name = name;
this.age = age;
}
sayHello() {
console.log(`Hello, my name is ${this.name} and I am ${this.age} years old.`);
}
}
class Phone {
constructor(model, color) {
this.model = model;
this.color = color;
}
call() {
console.log(`Calling from ${this.model} in ${this.color}.`);
}
}
이제 Person과 Phone을 조합하여 Contact 클래스를 만들어보자.
class Contact {
constructor(person, phone) {
this.person = person;
this.phone = phone;
}
introduce() {
this.person.sayHello();
this.phone.call();
}
}
위의 코드에서 Contact 클래스는 Person과 Phone을 조합하여 introduce() 메서드를 가지고 있다.
이제 밑의 예시처럼 Contact 클래스의 인스턴스를 만들어 사용할 수 있게 된 것이다.
const person = new Person('Hyein', 27);
const phone = new Phone('iPhone 13', 'white');
const contact = new Contact(person, phone);
contact.introduce(); // Hello, my name is Hyein and I am 27 years old. Calling from iPhone 13 in white.
예시 전체코드
class Person {
constructor(name, age) {
this.name = name;
this.age = age;
}
sayHello() {
console.log(`Hello, my name is ${this.name} and I am ${this.age} years old.`);
}
}
class Phone {
constructor(model, color) {
this.model = model;
this.color = color;
}
call() {
console.log(`Calling from ${this.model} in ${this.color}.`);
}
}
class Contact {
constructor(person, phone) {
this.person = person;
this.phone = phone;
}
introduce() {
this.person.sayHello();
this.phone.call();
}
}
const person = new Person('Hyein', 27);
const phone = new Phone('iPhone 13', 'white');
const contact = new Contact(person, phone);
contact.introduce(); // Hello, my name is Hyein and I am 27 years old. Calling from iPhone 13 in white.
'개발 > JavaScript' 카테고리의 다른 글
Set 객체: 값의 고유한 집합 저장소 (1) | 2023.12.17 |
---|---|
개인적인 This 정리 (1) | 2023.09.24 |
생성자 함수와 프로토타입의 차이? (0) | 2023.06.23 |
JS에서의 Boxing 처리 (0) | 2023.06.21 |
Array.prototype.reduce() (0) | 2023.05.07 |
@bokueyo :: 기록하는 습관
개발 블로그
포스팅이 좋았다면 "좋아요❤️" 누르기 !